package t09_thread_pool;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
public class ExecutorsExample {
public static void main(String[] args) {
// 스레드 풀 기능을 정의 해놓은 interface
final ExecutorService exe;
// thread pool을 생성하는 정적 메소드
// 초기 스레드 수 : 스레드풀이 생성될 때 기본적으로 생성되는 스레드 수
// 코어 스레드 수 : 스레드가 증가한 후 사용하지 않는 스레드를
// 스레드 풀에서 제거하지 않고 최소한으로 유지할 개수
// 최대 스레드 수 : 스레드 풀에서 생성 관리하는 최대 스레드 수
// newCachedThreadPool();
// 초기스레드, 코어 스레드 수가 0
// 일이 없는 스레드가 60초 이상 유지되면 스레드를 종료시키고 제거
// exe = Executors.newCachedThreadPool();
// 초기 스레드 수 : 0, 코어 스레드,최대 스레드는 매개변수로 지정
exe = Executors.newFixedThreadPool(3);
int coreCount = Runtime.getRuntime().availableProcessors();
System.out.println(coreCount); // 현재 운영체제의 사용가능한 프로세서개수
//익명구현객체 내에서 지역변수(여기서는 exe) 사용시 final이어야 함.
for(int i = 0; i < 16; i++) {
Runnable task = new Runnable() {
@Override
public void run() {
ThreadPoolExecutor tpe = (ThreadPoolExecutor)exe;
// 스레드 풀에서 관리하고 있는 스레드 수
int poolSize = tpe.getPoolSize();
String threadName = Thread.currentThread().getName();
System.out.println("[총 스레드 수 : "+poolSize+"] 작업 스레드 이름 : "+threadName);
int i = Integer.parseInt("일");
}
}; // task 정의 끝
//스레드 풀에 작업 등록
// execute : 다 쓴 스레드를 버림 - 예외가 처리되지 않음 - 오류가 생기면 처리할 수 없음.
//exe.execute(task);
// submit : 예외 처리 가능하기 때문에 기존 스레드를 재활용
Future<?> future = exe.submit(task);
try {
//오류는 던진다
future.get();
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
} // 반복문 종료
//이미 등록된 작업은 실행하고, 새로운 작업은 추가하지 않음.
exe.shutdown();
/*
exe.execute(()->{
System.out.println("추가된 작업");
});
*/
try {
// 완료되지 않은 작업이 있다고 하면 해당 시간만큼 대기
exe.awaitTermination(10, TimeUnit.SECONDS); //10초
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("wait 완료");
//스레드 풀의 모든 스레드를 즉시 종료
exe.shutdownNow();
System.out.println("프로그램 종료");
} // 메인 종료
}
package t09_thread_pool;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class ResultCallableExample {
public static void main(String[] args) {
int core = Runtime.getRuntime().availableProcessors();
System.out.println("컴퓨터 논리 프로세서 개수 : "+ core);
ExecutorService exe = Executors.newFixedThreadPool(core);
System.out.println("[작업 처리 요청]");
Callable<Integer> call = new Callable<Integer>() {
@Override
public Integer call() throws Exception {
int sum = 0;
for(int i = 1; i<= 100; i++) {
sum += i;
}
Thread.sleep(2000);
return sum;
}
};
Future<Integer> future = exe.submit(call);
try {
System.out.println("[ blocking ]");
int sum = future.get();
System.out.println("[작업처리결과 : "+sum+"]");
System.out.println("작업처리완료");
} catch (InterruptedException e) {
System.out.println("외부 호출에 의해 스레드 종료 : " + e.getMessage());
} catch (ExecutionException e) {
System.out.println("작업에서 발생한 예외 : "+ e.getMessage());
}
exe.shutdownNow();
}
}
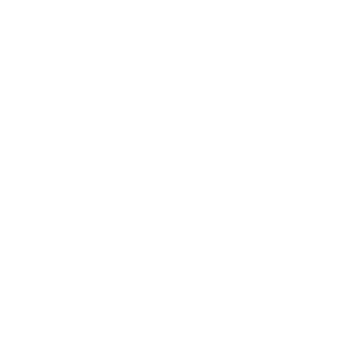
package n0_url_inet_address;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.InetAddress;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.UnknownHostException;
public class InetAddressURLExample {
public static void main(String[] args) {
try {
// 내 컴퓨터 호스트 정보
InetAddress address = InetAddress.getLocalHost();
System.out.println(address);
// 내 아이피 주소
System.out.println(address.getHostAddress());
// 컴퓨터 이름
System.out.println(address.getCanonicalHostName());
InetAddress naver = InetAddress.getByName("www.naver.com");
System.out.println("naver : "+naver.getHostAddress());
InetAddress[] iar = InetAddress.getAllByName("www.naver.com");
for(InetAddress remote : iar) {
System.out.println("naver.com IP 주소 : " + remote.getHostAddress());
}
// https://comic.naver.com/webtoon/list?titleId=808994
URL url = new URL(" https://comic.naver.com/webtoon/list?titleId=808994 ");
System.out.println(url);
System.out.println(url.getPath());
System.out.println(url.getProtocol());
System.out.println(url.getHost());
System.out.println(url.getPort()); // 포트번호 따로 지정하지 않아서 -1로 나옴
System.out.println(url.getQuery());
System.out.println(url.getFile());
InputStream is = url.openStream();
InputStreamReader isr = new InputStreamReader(is);
BufferedReader reader = new BufferedReader(isr);
File file = new File("naver.html");
FileWriter writer = new FileWriter(file);
BufferedWriter bw = new BufferedWriter(writer);
String isLine;
while((isLine = reader.readLine() )!= null) {
System.out.println(isLine);
bw.write(isLine);
bw.newLine();
}
bw.flush();
bw.close();
reader.close();
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (MalformedURLException e) {
// 전달한 URL이 잘못된 프로토콜일 수 있다.
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
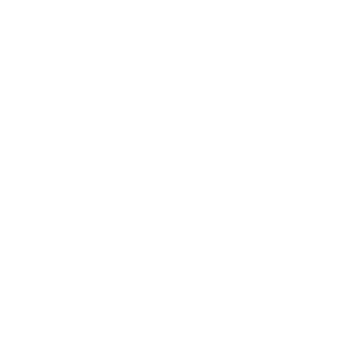
tcp/ip이용한 서버 프로그램생성
package n2_chat.client;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.InetSocketAddress;
import java.net.Socket;
import java.util.Scanner;
public class ClientExample {
Scanner sc = new Scanner(System.in);
// server와 연결 정보를 저장
Socket socket;
public ClientExample() {
startClient();
}
// server에 메세지 전달
public void send(String message) {
OutputStream os = null;
byte[] bytes = message.getBytes();
try {
os = socket.getOutputStream();
os.write(bytes);
os.flush();
System.out.println("데이터 발신 완료");
} catch (IOException e) {
System.out.println("서버와 연결 끊김");
try {
if(os != null) os.close();
} catch (IOException e1) {
e1.printStackTrace();
}
stopClient();
}
}
// server에서 전달된 데이터 수신
public void receive() {
Thread t = new Thread(()->{
while(true) {
InputStream is = null;
byte[] bytes = new byte[100];
try {
is = socket.getInputStream();
int readBytes = is.read(bytes);
String message = new String(bytes,0,readBytes);
System.out.println(message);
} catch (IOException e) {
System.out.println("서버와 연결 종료");
stopClient();
break;
}
}
});
t.start();
}
// client 시작
public void startClient() {
try {
socket = new Socket();
socket.connect(new InetSocketAddress("10.100.205.231",5002));
System.out.println("연결 정보 : "+ socket.getRemoteSocketAddress());
// 서버에서 전달되는 메세지 수신 - receive()
receive();
while(true) {
System.out.println("메세지 입력 : ");
String message = sc.nextLine();
send(message);
}
} catch (IOException e) {
System.out.println("서버와 통신 안됨 : "+ e.getMessage());
stopClient();
return;
}
}
// client 안전하게 종료 - 자원해제
public void stopClient() {
System.out.println("연결 해제");
if(socket !=null && !socket.isClosed()) {
try {
socket.close();
} catch (IOException e) {}
}
}
public static void main(String[] args) {
new ClientExample();
}
}
package n2_chat.server;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.List;
import java.util.Vector;
public class ServerExample {
ServerSocket serverSocket;
static List<Client> clients = new Vector<>();
//기본 생성자 호출 시 서버 시작
public ServerExample() {
startServer();
}
// server 포트를 할당받아 서버를 시작하고
// client의 연결을 담당할 메소드
public void startServer() {
try {
// serverSocket = new ServerSocket(5002);
serverSocket = new ServerSocket();
// 포트 할당 받기 전까지 blocking
// 내 컴퓨터 아이피 주소 == localhost == 123.0.0.1
serverSocket.bind(new InetSocketAddress("10.100.205.177",5002));
System.out.println("[ Server Open]" + serverSocket.getLocalSocketAddress());
} catch (IOException e) {
e.printStackTrace();
}
while(true) {
try {
System.out.println("[Client 연결 대기중...]");
Socket client = serverSocket.accept();
InetSocketAddress isa =
(InetSocketAddress)client.getRemoteSocketAddress();
String host = isa.getHostName();
System.out.println("연결수락 : "+host+"]");
// Client 정보 저장
clients.add(new Client(client));
System.out.println("연결된 클라이언트 수 : " + clients.size());
} catch (IOException e) {
System.out.println("서버 종료 : "+e.getMessage());
stopServer();
break;
}
}
}
// 안전하게 server가 종료될 수 있도록 사용된 자원 해제
public void stopServer() {
System.out.println("서버 종료");
try {
for(Client client : clients) {
// socket이 close되지 않았다면
if(!client.socket.isClosed()) {
client.socket.close();
}
}
if(!serverSocket.isClosed()) {
serverSocket.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
new ServerExample();
}
}
package n2_chat.server;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.Socket;
// socket으로 연결된 client의 정보를 저장하고 송수신을 담당할 class
public class Client {
//연결된 client socket 정보를 저장하는 field
Socket socket;
public Client(Socket socket) {
this.socket = socket;
receive();
}
// client에 메세지 전달
public void send(String message) {
OutputStream os = null;
byte[] bytes = message.getBytes();
try {
os = socket.getOutputStream();
os.write(bytes);
os.flush();
} catch (IOException e) {
System.out.println("Clinet 통신 안됨");
try {
if(os!=null) {os.close();}
} catch (IOException e1) {}
try {
if(socket!=null && !socket.isClosed())socket.close();
} catch (IOException e1) {}
ServerExample.clients.remove(this);
}
}
// client에서 출력된 데이터 수신
public void receive() {
// 반복하면서 client에서 출력된 데이터를 수신
System.out.println("receive 호출");
Thread t = new Thread(new Runnable() { //accept다음에 read한다고 메인스레드 멈춰있기때문에 스레드 추가
@Override
public void run() {
while(true) {
InputStream is = null;
byte[] bytes = new byte[100];
try {
is = socket.getInputStream();
int readCount = is.read(bytes);
// 발신자 정보 ip:port
String sender = socket.getInetAddress().getHostAddress()+":"+socket.getPort();
String message = new String(bytes,0,readCount);
message = sender + ":" + message;
System.out.println("수신처리 완료 : "+message);
for(Client c : ServerExample.clients) {
c.send(message);
}
} catch (IOException e) {
System.out.println("Client 통신 안됨 -");
try {
if(is != null) {is.close();}
} catch (IOException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
try {
if(!socket.isClosed()) socket.close();
} catch (IOException e1) {
e1.printStackTrace();
}
ServerExample.clients.remove(Client.this);
//receive 스레드 종료
break;
}
}// while end
}//run end
});//thread end
t.start();
}
}